BotsCrew Bot Framework
Create a bot in Java in 15 minutes
When we had first began Botscrew, a chatbot development company, we were faced with the problem of not having the appropriate technology for building bots in Java. We took it upon ourselves to create for chatbots something simple and convenient while at the same time powerful yet scalable as Spring MVC for Web. After two years of working in chatbot field, trying out different approaches and patterns, we have finally created BotsCrew Bot Framework – technology we have been missing for the last two years.
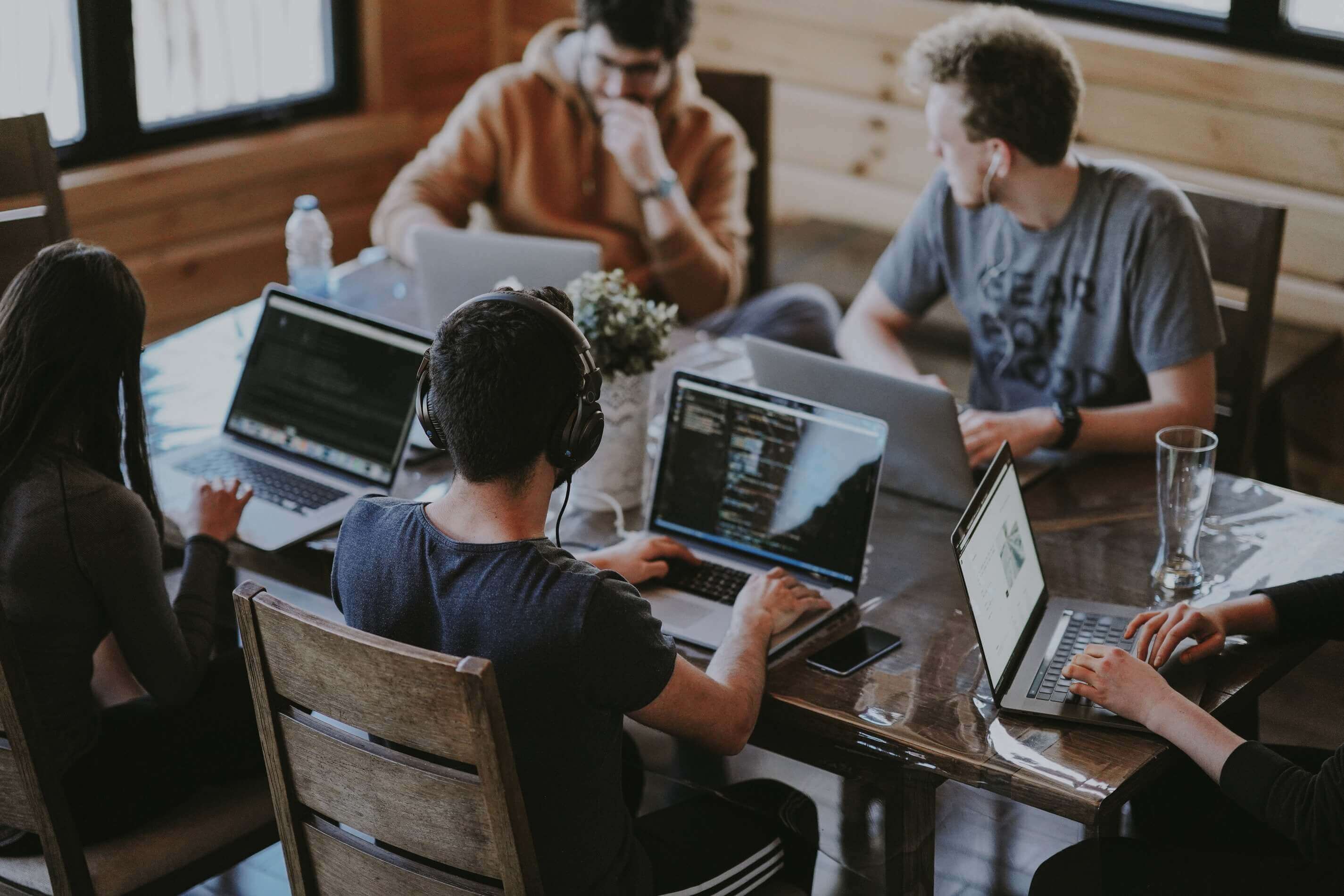
BotsCrew Bot Framework – the lightweight Java framework that enables users to design their chatbot effortlessly. The framework eliminates the problems with specific messaging platforms that may occur while developing a bot. Bot Framework allows you to build well-structured conversations with users by helping to create a logical and engaging flow that is divided into relevant blocks. The framework lets you get your chatbot up and running and is simple to use even for those with no experience with chatbots.
Bot Framework is based on Spring Boot architecture and comes as a set of modules for different purposes.
Here are a few of the modules:
Bot-framework-core – this main module supports multiplatform, contains logic and defines architecture for working with conversational flows.
Bot-framework-messenger – acts as the connector with Facebook Messenger platform, contains logic for receiving and sending messages, as well as getting user profile info, etc.
Bot-framework-NLP – connects your chatbot to NLP providers and gives you ability to manage complex text based flows. For now, this module supports both versions of DialogFlow API (other providers are coming soon)
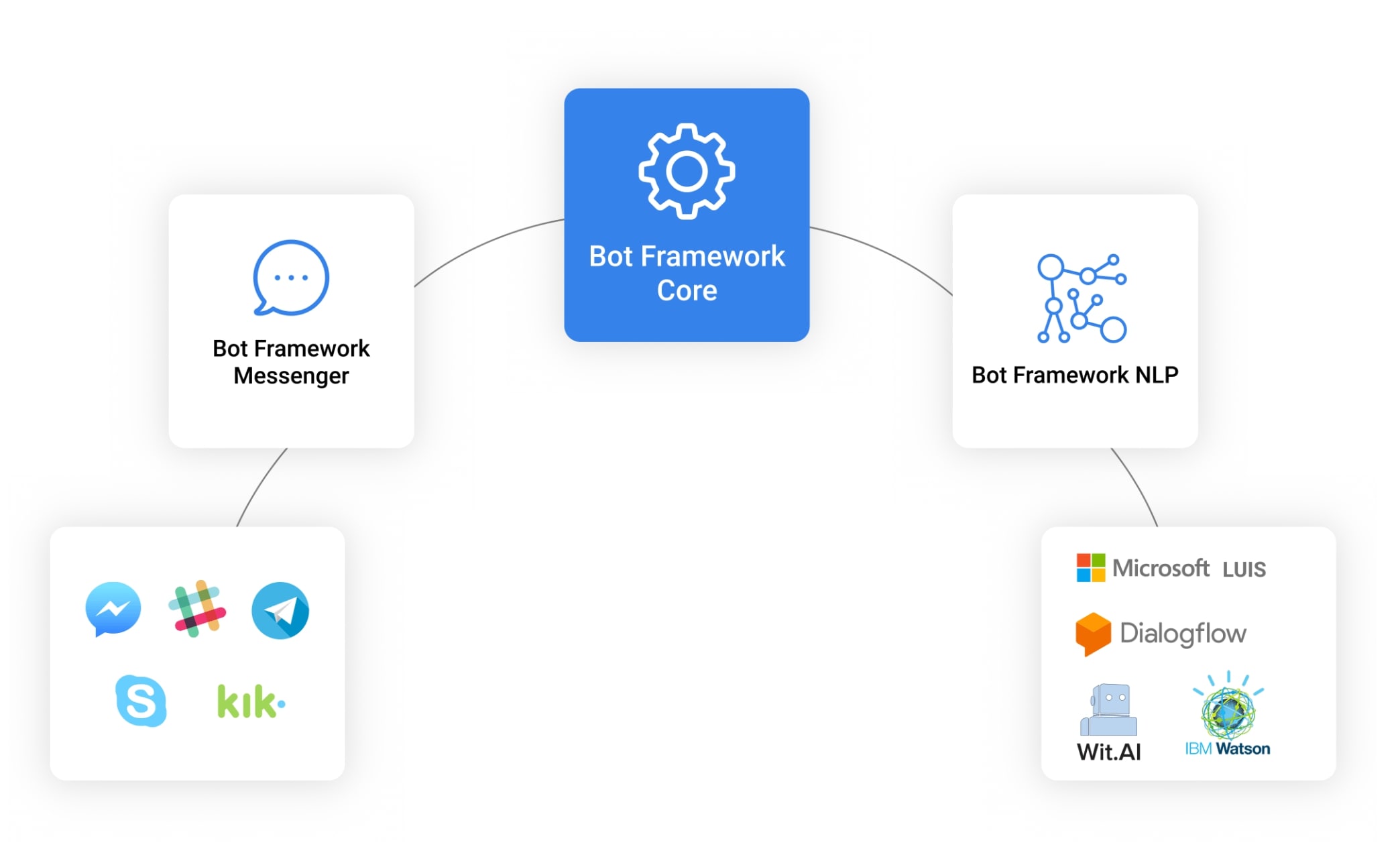
What Are The Benefits Of Bot Framework?
Fast
Bot Framework enables you to have a quick start with your chatbot in as little as 15 minutes
Conversational flow support
Bot Framework simplifies the conversational flow creation and makes it more structured and appealing.
No black box issue
Bot Framework is just a set of libraries allowing you to run it wherever you would like. Additionally, no one else will have access to your users and data.
Free
Take and use it as you please. The only “payment” we would be happy to receive from you is feedback from using Bot Framework.
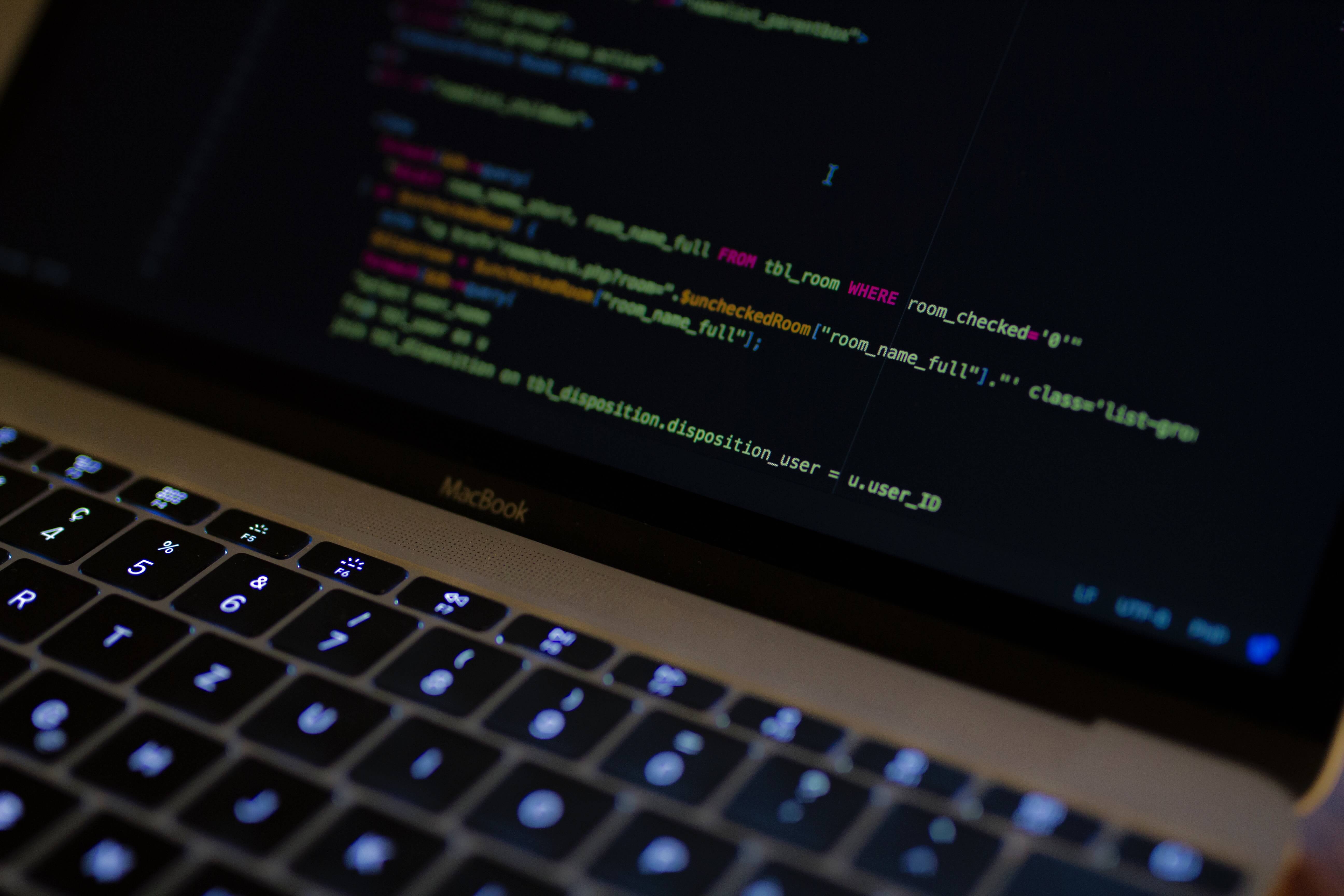
Talk is cheap. Show me the code.© Linus Torvalds
Let’s have a look at some code. We will now create a simple project to show you how the Bot Framework works.
Let’s design a taxi chatbot for Facebook Messenger.
For example, if we would like for our conversation to look the following way:
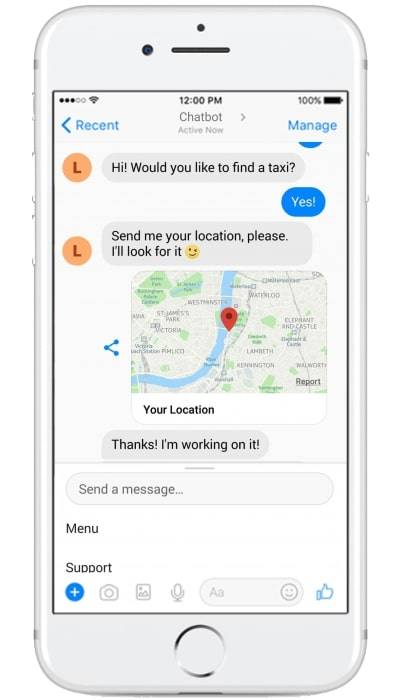
How would we do that?
First of all, let’s have a look in Facebook Messenger docs.
You can skip this step and go ahead if you are already familiar or just don’t like using docs.
We will start with creating a basic Spring Boot project. If you have worked with it before, you will know what to do. If not, take a look here: http://spring.io/projects/spring-boot
After that, add dependencies for the needed modules:
Bot Framework Core
<dependency> <groupId>com.botscrew</groupId> <artifactId>bot-framework--core-spring-boot-starter</artifactId> <version>1.0.0</version> </dependency>
Messenger Module
<dependency> <groupId>com.botscrew</groupId> <artifactId>bot-framework-messenger-spring-boot-starter</artifactId> <version>1.0.1</version> </dependency>
Now let’s create some classes where we will have our conversational logic. For example:
import com.botscrew.botframework.annotation.ChatEventsProcessor; import com.botscrew.botframework.annotation.Location; import com.botscrew.botframework.annotation.Postback; import com.botscrew.botframework.annotation.Text; import com.botscrew.messengercdk.model.MessengerUser; import com.botscrew.messengercdk.model.incomming.Coordinates; import com.botscrew.messengercdk.model.outgoing.builder.QuickReplies; import com.botscrew.messengercdk.model.outgoing.builder.SenderAction; import com.botscrew.messengercdk.model.outgoing.element.quickreply.PostbackQuickReply; import com.botscrew.messengercdk.model.outgoing.element.quickreply.QuickReply; import com.botscrew.messengercdk.service.Sender; import com.botscrew.messengercdk.service.Messenger; import org.springframework.beans.factory.annotation.Autowired; @ChatEventsProcessor public class MainHandler { private final Sender sender; private final Messenger messenger; @Autowired public MainHandler(Sender sender, Messenger messenger) { this.sender = sender; this.messenger = messenger; } @Text public void handleText(MessengerUser user) { String name = messenger.getProfile(user.getChatId()).getFirstName(); String message = "Hi " + name + "! Would you like to find a taxi?"; QuickReply yesReply = new PostbackQuickReply("Yes!", “FIND_TAXI”); sender.send( QuickReplies.builder() .user(user) .text(message) .addQuickReply(yesReply) .build()); } @Postback(“FIND_TAXI”) public void handleTaxiRequest(MessengerUser user) { sender.send(QuickReplies.builder() .user(user) .text("Send me your location, please. I'll look for it \uD83D\uDE09") .location() .build() ); } @Location public void handleLocation(MessengerUser user, Coordinates coordinates) { sender.send(user, "Thanks! I'm working on it!"); sender.send(SenderAction.typingOn(user)); //looking sender.send(user, "Unfortunately there are no cars for now\uD83D\uDE1E \n I will let you know if something appears", 5000); } }
Let’s go though this one by one and check what happens there:
@ChatEventsProcessor – this defines a class as a part of the conversational logic.
Sender – helps to send different types of messages to Facebook Messenger. It’s already configured, you will just need to autowire it.
Messenger – gives us a user profile. Also, you can find other useful methods there.
@Text – means this method will handle the text that users write.
@Postback(“FIND_TAXI”) – handles postback(button click) with FIND_TAXI payload.
@Location – handles user location.
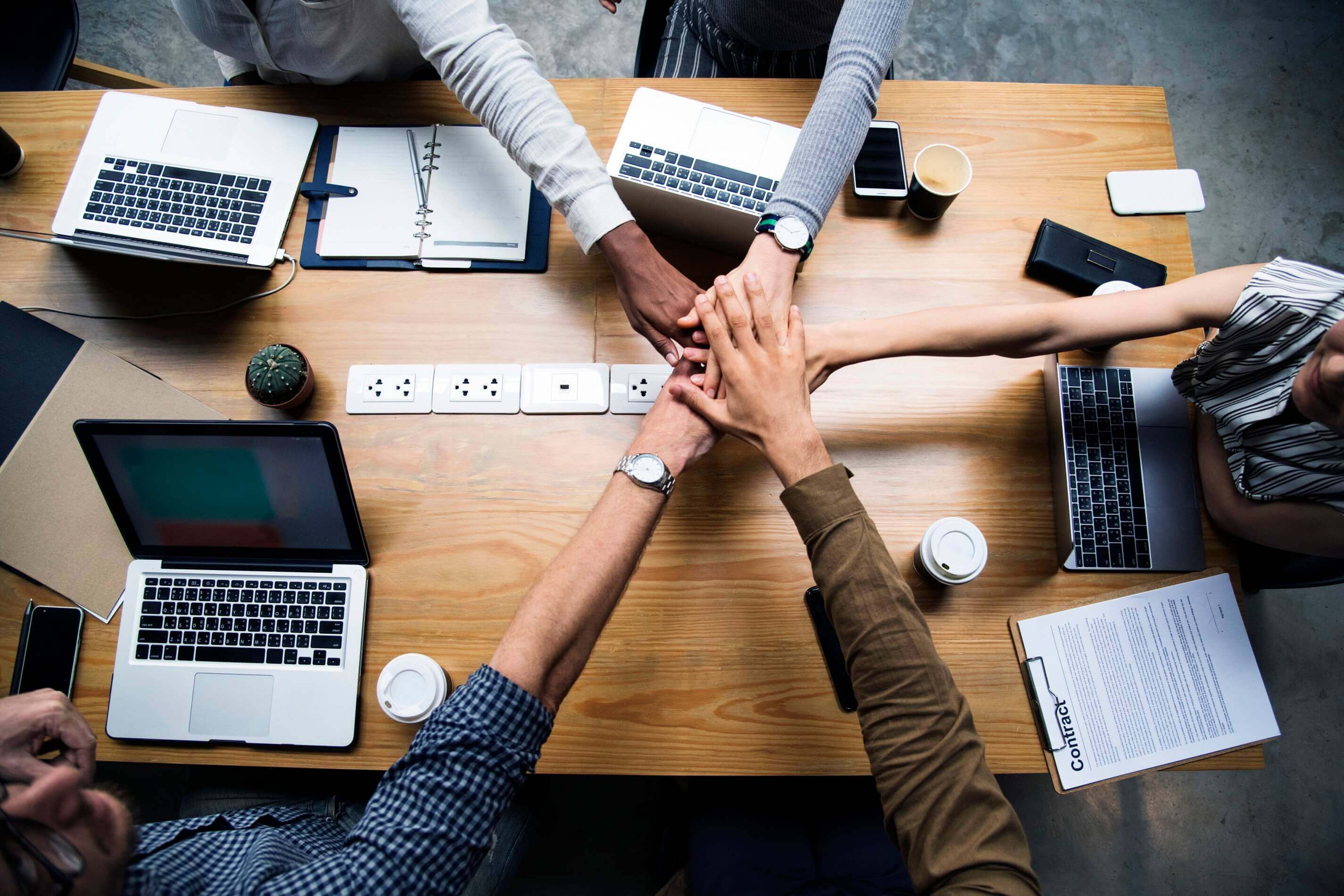
And, that’s it. We have completed this project. You need to connect your Facebook Page with this service and add a token for a page to the`application.properties.` file. (Property name is facebook.messenger.access-token)
After that, you can test to see how it works.
The only thing that you need to consider is the logic of the conversation. Bot Frameworks hides all the configurations from you, however, it is still easily customizable if necessary.
If you would like to know more about Bot Framework and its features have a look in docs or feel free to contact us. We will be glad to guide you and show some more of the advanced features.
Author: Vlad Hanzha